C언어에서의 동적 메모리 할당은 프로그래밍의 핵심 부분 중 하나입니다. 특히, malloc() 함수는 메모리의 동적 할당을 담당하여 프로그램의 효율성을 향상시킵니다. 이 블로그 포스팅에서는 malloc() 함수의 활용법뿐만 아니라, 동적 메모리 할당의 심화된 내용을 알아보고자 합니다.
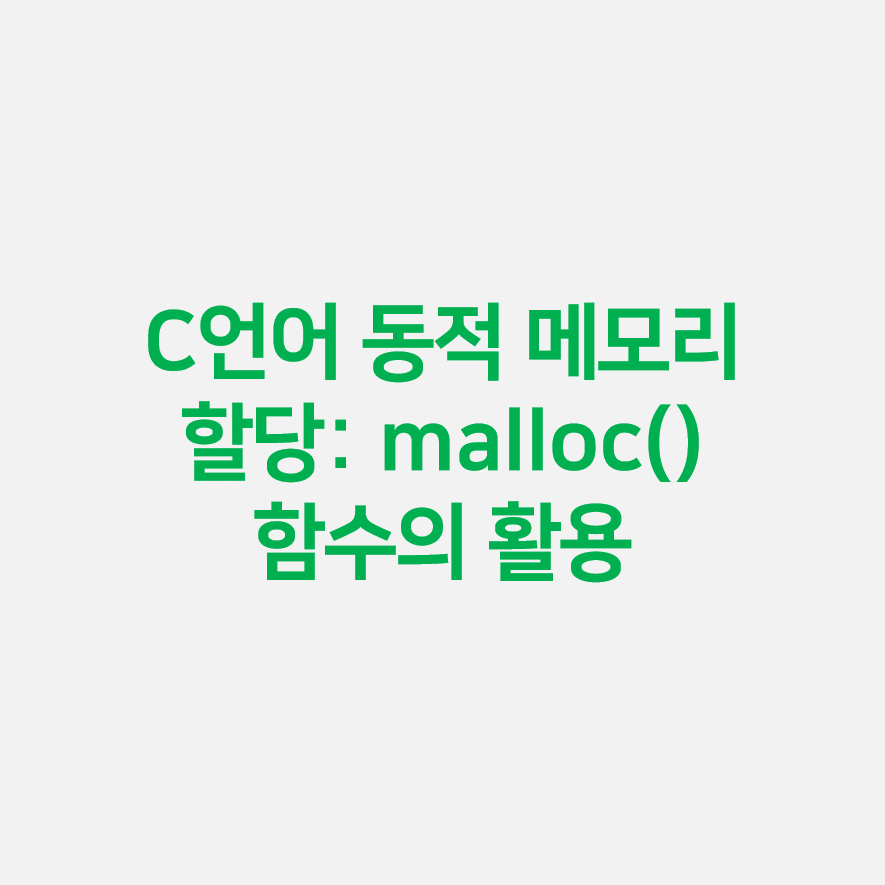
malloc() 함수 개념과 활용법
malloc() 함수는 C언어에서 동적 메모리 할당을 위한 주요 함수로, stdlib.h 헤더 파일에 선언되어 있습니다. 예를 들어, 프로그램에서 가변적인 데이터 크기를 처리해야 할 때 malloc() 함수를 사용하여 동적으로 메모리를 할당할 수 있습니다.
malloc() 함수 세부 사용법과 반환값
malloc()은 크기를 인자로 받아 해당 크기의 메모리를 할당하며, 성공 시 첫 번째 바이트를 가리키는 포인터를 반환합니다. 예를 들어, 정수 배열을 동적으로 할당하고 해당 배열을 활용하여 사용자로부터 숫자를 입력 받는 프로그램을 작성해보겠습니다.
int *arr;
int size;
printf("Enter the size of the array: ");
scanf("%d", &size);
arr = (int *)malloc(size * sizeof(int));
if (arr != NULL) {
printf("Memory allocation successful!\n");
// Further code to process the dynamically allocated array
} else {
printf("Memory allocation failed.\n");
}
size_t와 메모리 크기 지정의 활용
size_t는 부호 없는 정수로, 메모리 크기를 지정할 때 사용되며 항상 양수여야 합니다. 예를 들어, 구조체를 동적으로 할당할 때 구조체의 크기와 size_t를 결합하여 안정적인 메모리 할당을 구현할 수 있습니다.
struct Student {
char name[50];
int age;
};
struct Student *studentPtr;
size_t numStudents;
printf("Enter the number of students: ");
scanf("%lu", &numStudents);
studentPtr = (struct Student *)malloc(numStudents * sizeof(struct Student));
// Check for successful allocation and proceed accordingly
포인터와 malloc()의 관계 및 메모리 안정성
malloc()이 반환하는 void 포인터는 데이터 유형을 알지 못하므로, 프로그래머는 형변환을 통해 적절한 활용이 필요합니다. 예를 들어, 동적으로 할당한 메모리를 해제하기 전에 해당 메모리를 안전하게 활용하고 데이터를 채워넣는 프로그램을 작성해보겠습니다.
int *numbers;
int size;
printf("Enter the size of the array: ");
scanf("%d", &size);
numbers = (int *)malloc(size * sizeof(int));
if (numbers != NULL) {
printf("Memory allocation successful!\n");
// Populate the array with user input
for (int i = 0; i < size; ++i) {
printf("Enter number %d: ", i + 1);
scanf("%d", &numbers[i]);
}
// Further code to process the dynamically allocated array
} else {
printf("Memory allocation failed.\n");
}
동적 메모리 할당 예제
간단한 C 프로그램 예제를 통해 malloc()의 사용법을 실제 코드로 확인합니다. 예를 들어, 동적으로 할당한 배열을 정렬하고 결과를 출력하는 프로그램의 세부적인 코드를 다루어보겠습니다.
// Assume 'numbers' is a dynamically allocated array
// Sorting the array
qsort(numbers, size, sizeof(int), compare);
// Displaying the sorted array
printf("Sorted array: ");
for (int i = 0; i < size; ++i) {
printf("%d ", numbers[i]);
}
동적 메모리 해제 free()의 중요성
동적으로 할당한 메모리는 반드시 해제되어야 하며, 이를 위해 free() 함수의 활용법을 소개합니다. 예를 들어, 사용이 끝난 배열을 동적으로 할당된 메모리에서 해제하는 프로그램의 코드를 다루어보겠습니다.
// Assume 'numbers' is a dynamically allocated array
// Freeing the dynamically allocated memory
free(numbers);
마무리
이 블로그 포스팅에서는 C언어에서의 동적 메모리 할당과 malloc() 함수의 활용법을 더욱 심화시켜 다루었습니다. 실제 예제를 통해 각 단계를 자세하게 설명하고, 프로그램 개발 시의 다양한 상황에 대응하는 방법을 다뤘습니다. 메모리 해제의 중요성을 강조하며, 안정적인 프로그래밍을 위한 기초를 더욱 견고히 다지길 바랍니다. 더 많은 C 언어 관련 정보를 학습하고 싶다면, 관련 문서와 강의를 참고하시기 바랍니다. 감사합니다.
'컴퓨터 과학 > 데이터 구조' 카테고리의 다른 글
구조와 함수: 프로그래밍의 기본 (86) | 2023.11.26 |
---|---|
free() 함수를 활용한 동적 할당 메모리의 효율적인 해제 방법 (76) | 2023.11.25 |
알고리즘 성능평가의 핵심: 점근적 복잡도 (65) | 2023.11.23 |
알고리즘 성능평가의 핵심: 시간 복잡도와 실행 시간 측정 방법 (63) | 2023.11.22 |
데이터 구조의 이해: 선형과 비선형, 정적과 동적 데이터 구조 (57) | 2023.11.21 |